03: Introduction to Selenium WebDriver Run your first Selenium test steps
Run your first Selenium test steps.
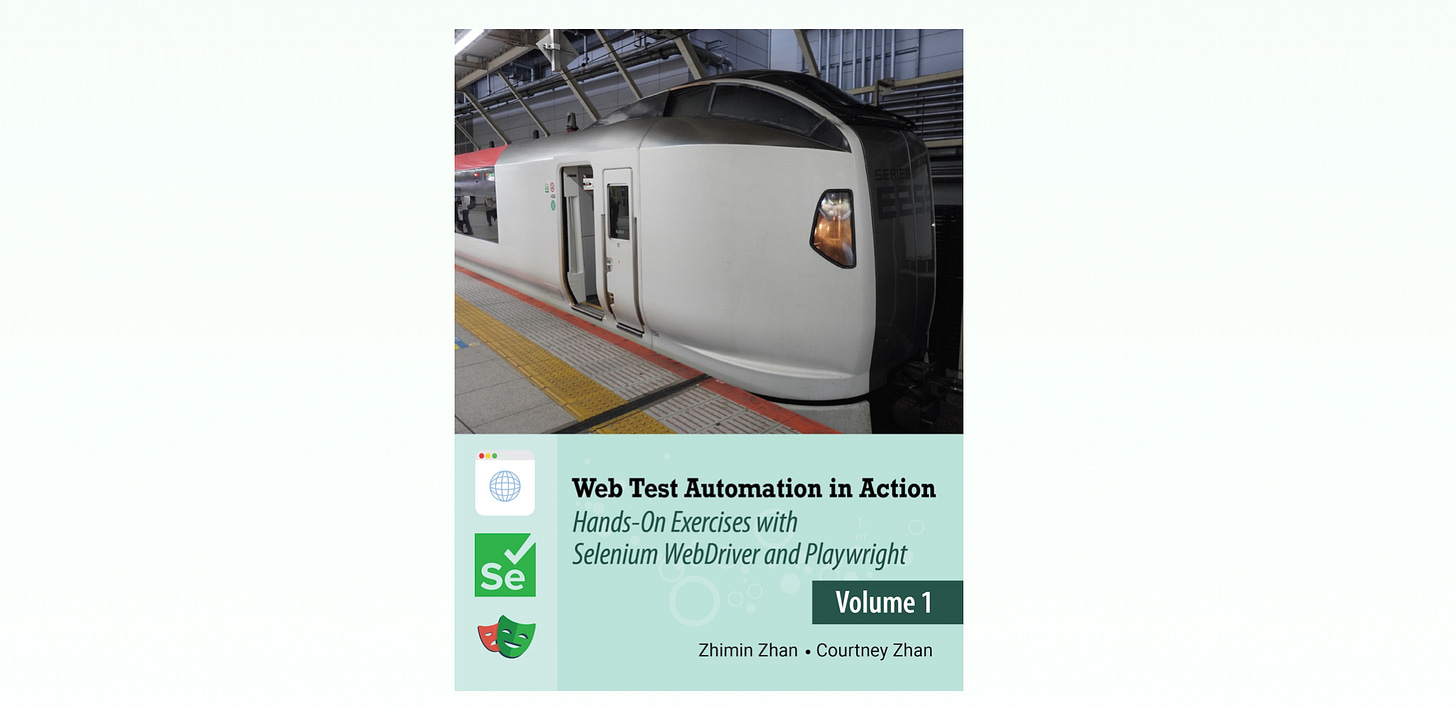
Learning Objectives
Basic Selenium WebDriver syntax
Three Selenium locators:
ID, NAME, LINK
Try out the Selenium test steps
TestWise’s debugging mode
(a fast way to try out selenium steps)
Selenium WebDriver’s syntax pattern
Selenium WebDriver is the easiest-to-learn automation framework because its syntax follows such a simple and intuitive pattern.
You may be reading this article (and others in this series) on smartphones. If so, I suggest stop and find 15 minutes to sit down in front of a computer: do the following exercises hands-on. In my opinion, the only way to learn and master test automation is by doing many exercises hands-on.
Step 1. Locate the control
Right-click a web control, e.g. a text field or link, in Chrome and select “Inspect”.
The HTML (introduced in session #2) for the selected control is highlighted on the right panel. In this example (above), the HTML for the user name text field (for users to enter a username) is
<input type="text" name="username" id="username" size="25">
Beginners might worry about the above syntax as they think they are not web developers. Please don’t worry. The HTML knowledge required by test automation engineers is actually quite limited.
Common Controls on a web page
There are a few web controls on web pages, and you are familiar with most of those and have used them already.
Link (a.k.a. hyperlink)
Click to navigate to another web page.Text Field (a.k.a. text box)
for users to enter textCheck box
Yes or No option.Button
An action, for example, submits a form (but is not limited to).Information controls
for users to read, not act on.
-h2
, heading 2 (there are alsoh1,h3,...)
-label
,span
-p
, paragraph
There are a few more types, HTML-wise, not much different.
Selenium WebDriver’s Locators
After understanding the controls (a.k.a. elements) on a web page, we will learn how to locate a specific element in Selenium. Do you still remember Selenium’s pattern: “Locate a web control, then drive it”?
The way to locate an element in Selenium is to use one of its eight locators. Let’s start with three simple ones.
ID
The HTML for the user name text field is
<input type="text" name="username" id="username">
The ID for this element is username
. To locate it in Selenium,
driver.find_element(:id, "username")
You may have some questions. Hold them for now, please. You just need to know that we can use “ID” (in HTML) to locate a specific web control.
2. Name
Besides ID
, we can use the element: NAME
.
<input type="text" name="username" id="username">
For the same control (user name text field), the below works too.
driver.find_element(:name, "username")
3. Link
This one is easy to understand: locating a hyperlink based on the displayed link text.
driver.find_element(:link, "Login")
We have covered 3 out of 8 Selenium locators now. They are not difficult, are they?
I will introduce the remaining locators in upcoming articles.
Step 2. Perform action on the located control
After locating a web control, what will be next? Perform an action on it. Think about what you can do with the above two controls:
Link: Click it
Text Field: Type text in it.
How will they be done in Selenium?
1. click on a link
driver.find_element(:link, "Login").click
2. Send keys to a text field
driver.find_element(:id, "username").send_keys("agileway")
The selenium syntax is quite intuitive, isn’t it?
Note: some readers might think
type
(in Cypress) orfill
(in Playwright) more intuitive as an action for a text box. Wrong! You can “type” a piece of text, such as “Selelnium is much better than Cypress and Playwright”, but how will you type anEnter
orUp
key? Therefore,send_keys
is accurate,type
andfill
are not.
After all, WebDriver syntax was created by W3C experts after many rounds of review (took years). Comparativly, Cypress released its v1 without supporting Frames, what a joke! For more limitations of Cypress, check out my other article: Why Cypress Sucks for Real Test Automation? (Part 2: Limitations).
Run Selenium Scripts in TestWise
Now, let’s run the above Selenium scripts in TestWise.
In a one-on-one coaching session, I would have had TestWise ready for the attendees to try out the scripts (Apple calls it Playgrounds). If you haven’t installed TestWise, check out session #1 or the section in my daughter’s article: Set up, Develop Automated UI tests and Run them in a CT server on your First day at work. It only took about one minute to install the TestWise Ruby edition on Windows (watch the first 20s of the screencast below).
For readers using macOS or Linux, after Ruby & gems & chromedriver, install TestWise standard edition. It is not complex either.
If you are not to use TestWise, it is totally fine. All test scripts in this workbook are based on 100% free and open frameworks, and executable from the command line. You may continue this exercise with your preferred test tool, only with some of contents that will not apply. In this case, you can just focus on completing the test case and apply design/debugging practices with your chosen tool.
Get TestWise in Playgrounds mode
Launch TestWise
see session #01Click “Open Sample Project (web app)”
(see the screenshot below)Click
01_login_spec.rb
to open it
4. Right-click line 23 and select Run "[1] Can sign in OK"
to run this test case.
In fact, any line between under it "[1]...."
will do.
A Chrome browser window will launch. After test execution, this browser would remain open.
5. Select the line puts("Go to login page"
, right click and select Run Selected Scripts Against Current Browser
.
You will see that TestWise opens a new (if it does not exists already) file: debugging_spec.rb
6. Enter TestWise’s “debugging mode”
The “debugging mode” in Testing is different from the programmer’s way of using debuggers. It is far simpler. In TestWise, It is a “Playgrounds” mode to try out automated scripts.
Important note: the Chrome browser window must be kept open.
Try out automated test steps
Open (manually) the target web address (URL) in the Chrome browser launched by TestWise.
In this exercise, https://travel.agileway.netEnter one test line in the
debugging_spec.rb
betweenit "Debugging" do
andend
.
Let’s try clicking the Register
link.
it "Debugging" do
driver.find_element(:link, "Register").click
end
Click the blue triangle button on the toolbar to run it.
You will notice that the page in the Chrome browser window changed, i.e., the ‘Register’ link is clicked.
Type another step to click the Login
link.
it "Debugging" do
driver.find_element(:link, "Register").click
driver.find_element(:link, "Login").clickend
Run it again.
Tip: I suggest moving the chrome window (making it smaller) and TestWise side by side.
Astute readers might notice that the “Register” link got clicked again. That is correct. How to just click the ‘Login’ link but not delete the one above?
Knowledge Point: Comment in code/scripts
Make one or more test steps as comments so that they won’t be run. The comments in code/scripts are ignored by computers but may help human beings (understand or keep statements that are temporarily not applicable). To do that in Ruby: add the #
in the front of the line.
it "Debugging" do
# driver.find_element(:link, "Register").click
driver.find_element(:link, "Login").click
end
Try it out.
The page shown in the browser shall be the Login page.
Let’s fill in a user name.
it "Debugging" do
# driver.find_element(:link, "Register").click
driver.find_element(:link, "Login").click
driver.find_element(:id, "username").send_keys("agileway")
end
Run the above in TestWise ‘debugging mode” (again).
The text was entered in the textbox.
Please feel free to try more, such as
enter another username
enter a password
click another link
FAQ
What are the differences, in terms of execution, between TestWise’s debugging and normal mode?
In TestWise’s debugging mode, you don’t need to launch a new browser window. You will continue with the lastly opened browser window. There are many benefits of doing that. For now, you have surely noticed one: it is faster!
Can I manually navigate to another site (or page) and then continue running new/existing test steps in TestWise debugging mode?
Yes, as long as TestWise is still attached to the chrome window. Note, TestWise is only able to attach to the most recent Chrome Window launched by TestWise.
Can I use this debugging mode in Visual Studio Code or other tools?
Maybe. The technique is public (confident programmers can read the
test_helper.rb
, Searching--remote_debugging_port
). For more, check out my other article “My Innovative Solution to Test Automation: Attach test execution to the existing browser”.
However, to my knowledge, there is no testing tool to support it as what TestWise does. By the way. Visual Studio Code is a powerful programmer’s editor, not a testing tool. It is possible to develop an extension like TestWise’s debugging mode though.